Runtime And Compile Time Polymorphism
Polymorphism is the process where the same action can be performed in a number of different ways. Java supports 2 types of polymorphism – Compile time and runtime.
Compile time polymorphism
Java supports compile-time polymorphism via method overloading.Method overloading allows us to define two or more methods with the same name within a class but with different parameter declarations.
Consider the following code snippet:
This class has 4 methods with the name add. All four add methods differ in the parameters, so there is no compilation error. 1st add method does not have any parameters, the 2nd add method takes two integer parameters, the 3rd add method takes one integer parameter and the 4th add method takes two double parameters. So the add method is said to be “overloaded” four times.
Since the same action can be performed in a number of different ways, method overloading is an example of polymorphism. It is known a compile-time polymorphism because the version of an overloaded method to be invoked is determined at compile time by the compiler based on the parameters being passed in. So even before Java executes the program, the compiler determines which version of the method to invoke.
Runtime polymorphism
Java achieves Runtime polymorphism via method overriding. In a class hierarchy, when a method in a subclass has the same name and type signature as a method in its superclass, then the method in the subclass is said to override the method in the superclass.
Consider the following code:
The main difference between Static and Dynamic Polymorphism is that Static Polymorphism is a type of polymorphism that resolves at compile time while Dynamic Polymorphism is a type of polymorphism that resolves at run time. OOP is a popular software paradigm which allows programmers to model the real world scenarios as objects. Polymorphism is a major pillar of OOP.
Car and Bicycle are both subclasses of Vehicle. Both have a method called printVehicleType.
Now consider the following code:
First, we are creating a vehicle object. We are then creating a car object and assigning it to vehicle. We are then invoking the printVehicleType method using the vehicle variable. This will invoke the method in the car class.
Next, we are creating a bicycle object and assigning it to vehicle. We are then invoking the printVehicleType method again using the vehicle variable. This will invoke the method in the bicycle class.
This is how Java achieves runtime polymorphism. Since the same method call i.e. vehicle.printVehicleType prints different values based on the object assigned to it, this is an example of polymorphism. It is called runtime polymorphism because the version of the overridden method to be invoked is determined at runtime.
Related blog:
Polymorphism is Greek for 'many forms'. There are two types ofpolymorphism: static and dynamic. Static polymorphism occurs atcompile time and is also known as compile time polymorphism.Dynamic polymorphism occurs at runtime and is also known as runtimepolymorphism.
Runtime polymorphism is a primary feature of the object-orientedprogramming paradigm. We achieve runtime polymorphic behaviour bydefining virtual methods in a base class which derived classes canthen override in order to provide more specialised implementations.Whenever we invoke one of these methods, the most-specialisedoverride is executed automatically. In other words, polymorphicobjects will behave according to their runtime type even when theruntime type cannot be determined at compile time.
What do you meant by Runtime Polymorphism?
Runtime polymorphism is also called as method overriding, late binding or dynamic polymorphism. It is when a method in a subclass overrides a method in its super class with the same name and signature.
What is Difference between dynamic polymorphism and static polymorphism with example?
In static polymorphism is exhibited at compilation time, where as dynamic exhibited at runtime..
Why run time polymorphism is dynamic and compile time polymorphism is static?
Runtime prolymorphism means overriding compiletile polymorphism means overloading
Types of polymorphism in oops?
two types are compiletime and runtime polyymorphism
What is the difference between compile time and run time polymorphism?
Runtime prolymorphism means overriding compiletile polymorphism means overloading
What is the difference between run time polymorphism and compiler polymorphism?
The simple answer is that compile-time polymorphism occurs at compile time while runtime polymorphism occurs at runtime. The actual answer is that compile-time polymorphism results in the compiler generating source code on your behalf while runtime polymorphism relies on function pointers or virtual methods to determine the next instruction at runtime. Compile-time polymorphism therefore applies to template functions and classes since that is the only way the compiler can generate source code on your behalf… Read More
How polymorphism can be implemented in c plus plus?
In C++, compile-time polymorphism is achieved through the use of template metaprogramming while runtime polymorphism is achieved through virtual functions.
What is dynamic method dispatch in java?
dynamic method dispatch is a technique by which a call to a overridden method is solved at runtime rather than compile time.this is how java implements runtime polymorphism.
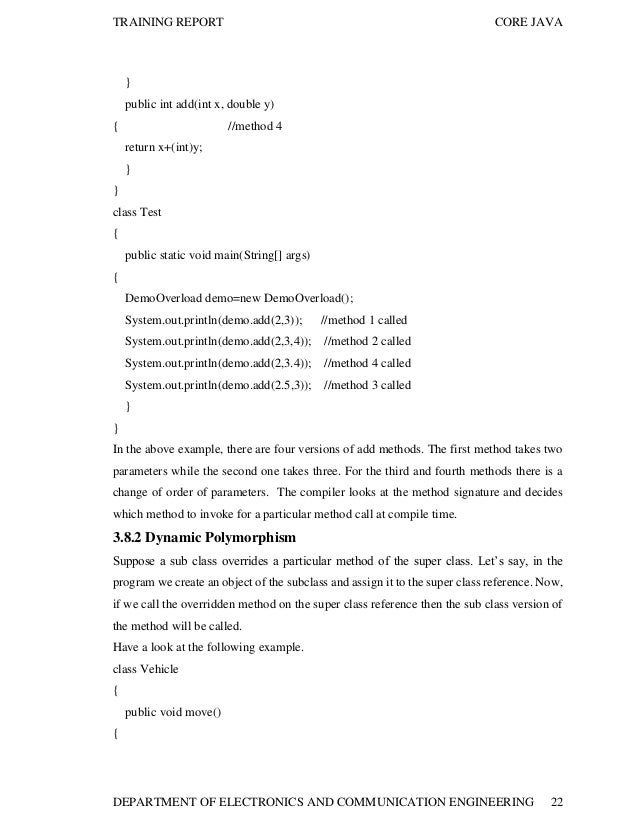
Is late binding and dynamic binding related to polymorphism?
Late binding and dynamic binding are related to runtime polymorphism. By contrast, compile time polymorphism is known as static binding. Template functions and classes are examples of static binding because the exact type can be determined at compile time.
Define polymorphism in c plus plus?
There are two types of polymorphism: static and dynamic. Static polymorphism applies to templates (both functions and classes), where the compiler generates the specific function or class according to the types you use with the template. Dynamic polymorphism is achieved at runtime through virtual functions. This ensures that the most derived implementation of a function is called regardless of which base class interface is used to invoke the function.
What is the different between polymorphism and dynamic binding?
In computer science, polymorphism refers to a computer language's ability to process objects according to their data type. Dynamic binding on the other hand, is a mechanism wherein the method used on an object is being looked up by name at runtime.
What is polymorphism in Visual c plus plus?
Polymorphism in VC++ is the same as polymorphism in C++ itself. When you implicitly call a virtual method of a base class or one of its derivatives, then you rightly expect the most-derived override to execute instead, even when the runtime type is completely unknown to the caller and cannot be determined at runtime let alone compile time. You get that for free simply by overriding the known virtual methods of the base class, and… Read More
How does virtual function support run time polymorphism?
Virtual functions are used to suport runtime polymorphism.In C++,if we have inheritance and we have overridden functions in the inherited classes,we can declare a base class pointer and make it to point to the objects of derived classes.When we give a keyword virtual to the base class functions,the compiler will no do static binding,so during runtime ,the base class pointer can be used to call the functions of the derived classes.Thus virtual functions support dynamic… Read More
When runtime error occurs?
What are the different types of polymorphism?
Implicit Parametric Polymorphism Subtype Polymorphism Explicit Parametric Polymorphism
Why do you need runtime polymorphismis inheritance necessary for it?
Dynamic Polymorphism In Java
Runtime polymorphism is required because accessing the runtime type of an object is expensive. And yes, inheritance is required for it because polymorphism is enabled through virtual functions. It is not the programmer's responsibility to determine the runtime type of an object because that information should be provided by the object's own virtual table. Thus when you implicitly invoke a virtual method of the base class, you rightly expect most-derived method to be executed, even… Read More
What is run time polymorphism c plus plus?
Runtime polymorphism is the means by which we can write generic code that invokes specific behaviour without the need to employ expensive runtime type information. That is, if we design several classes that share common features, we can percolate that shared functionality up to a common base class and work with those generic classes rather than the more specific classes. By declaring all the common functionality as virtual methods of the base class, and implementing… Read More
Difference between operator overloading and polymorphism?
Operator overloading simply means to provide a new implementation for an existing operator. Polymorphism is a function of inheritance whereby it is not necessary to know the runtime type of an object so long as you know the generic type. The virtual table ensures you gain specific behaviour by calling the generic type's virtual methods.
Polymorphism in coelentrates?
What are Principles of object oriented programming language?
The principles of object-oriented programming are: Encapsulation (hiding interface from implementation) Inheritance (classes of objects can inherit functionality from parent classes) Polymorphism (objects respond to methods based on their runtime-type).
Types of polymorphism?
Describe about the polymorphism in coelenterates?
does polymorphism is a characteristics feature of coelentrates
Compile Time And Runtime Polymorphism In Java
Is overloading a example of polymorphism?
What is dynamic polymorphism in c plus plus?
Dynamic polymorphism is polymorphism that is resolved only at runtime. Consider the following example: class A{ data members; methods; void display(); } class B : public A{ data members; methods; void display(); } int main(){ A obj; B obj1; A *ptr; ptr = obj; obj->display();//Here the display defined in A is called ptr = obj1; obj1->display();//Here the display defined in B is called return 0; } As seen in the above example, the display function… Read More
Where to use polymorphism?
Polymorphism is used whenever you wish to achieve specific behaviour from a generic object, where the object's actual type may not be known or would be impossible to determine at compile time. By declaring virtual methods in the generic type (the base class), and overriding them in the derived type, you ensure that the derived object does 'the right thing' regardless of its actual type, and regardless of whether the method is called directly or… Read More
Do hydra exhibit polymorphism?
yes they exhibit polymorphism. they have zooids and hydroids
Is method overloading is polymorphism?
What is Difference between dynamic polymorphism and static polymorphism?
Static polymorphism is used the concept of early binding or we can say compile time binding where as dynamic polymorphism used the concept of late binding or run time binding.
Is constructor overloading a polymorphism concept?
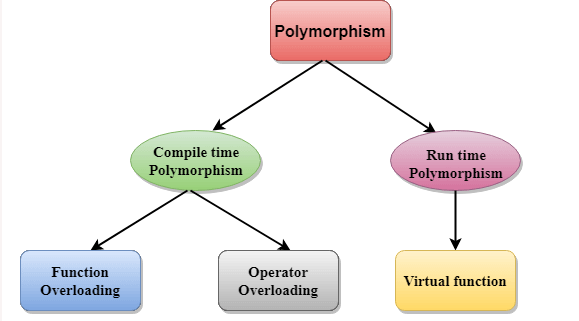
I think constructor overloading is the concept of polymorphism.
What kind of drugs commonly has polymorphism?
Polymorphism is common with barbiturates, steroids, and sulphonamides.
What is polymorphism and its types?
Polymorphism means multiple form of a function, variable or object. In Computer Science, polymorphism is a programming language feature that allows values of different data types to be handles using a common interface. There are three types : Ad-Hoc Polymosphism, Parametric Polymorphism, Subtype/Inclusion Polymorphism. Source: Wikipedia.
What is mean by polymorphism in java?
polymorphism is more than one name with different forms
How can one detect polymorphism by genetic marker?
One can detect polymorphism by genetic marker using single-nucleotide polymorphism which is able to even tell mutation of a gene.
How is Polymorphism used in C plusplus?
Polymorphism allows you to treat specific objects generically. For instance, if you have a collection of shapes such as circles, squares and triangles, you can treat them generically as being a type of shape, simply by deriving them from a common base class. The common base class can be a standalone class (from which objects can be instantiated) or it can be an abstract class (from which only derivatives can be instantiated). So long as… Read More
What is the benefit of run time polymorphism in c plus plus?
The benefit of runtime polymorphism is that derived objects behave correctly even when you cannot know the exact type of the derivative in advance; all you know for certain is what kind of base class it is derived from. This ensures that even when other programmers derive new objects from the base class (which you cannopt possibly know about in advance), they will behave according to their actual type, simply by overriding the virtual methods… Read More
How do you distinguish polymorphism and allotropy?
Polymorphism and Allotropy are same thing. Polymorphism is used for compounds and the allotropy is reserved for elements. You can go for XRD to check the crystal structure and their composition to distinguish.
What is run time polymorphism?
In run time polymorphism compiler doesn't know about way of execution of program that mean decide way of execution at run time while in compile time polymorphism compiler know about the way of execution of program. Example : compile time polymorphism -- method overloading run time time polymorphism -- method overriding
How do you implement run time and compile time polymorphism?
Run time polymorphism maybe achieved by implementing a particular interface among different class hierarchies, or using the same delegate from different objects. (delegate maybe thought as an interface to invoke methods with different names but with the same parameters. These methods may or may not be of the same object/class) Compile time polymorphism is achieved by class inheritance or implementing an interface. Run time way means the actual implementing class is UNKNOWN (uncertain) at compile… Read More
How Can you Implement Polymorphism in Java?
By implementing polymorphism we need to use overloading and overriding Technics in program.
Is Interfaces in Java a kind of polymorphism?
No. Interfaces in Java are a construct to get polymorphism ( subtype polymorphism ) working in Java, but they are not a 'kind' of polymorphism. In polymorphism happens when two objects respond to the same message ( method call ) in different way ( hence poly -> many, morphism -> way or shape : polymorphism -> many ways). In Java to be able to send the same message to two different objects you have to… Read More
What is drug polymorphism?
Drug polymorphism is a customerテ不 variation in response to a drug is by gender, body composition, size and age. The first polymorphism was first labelled over 40 years ago.
Define compile time polymorphism with short examples?
compiler can decide which form of the object should be invoked during compile time.this type of polymorphism is know as compile time polymorphism
Why you use polymorphism?
Polymorphism allows you to use the same function name but with different arguments (of different type).
Polymorphism and single inhertance program in java?
Polymorphism means The ability to take more than on form
What is polymorphism in c plus plus?
Polymorphism is the ability to use an operator or function in different ways. Polymorphism gives different meanings or functions to the operators or functions. Poly, referring to many, signifies the many uses of these operators and functions. A single function usage or an operator functioning in many ways can be called polymorphism. Polymorphism refers to codes, operations or objects that behave differently in different contexts.
If you make a exe in vbnet can you run it on a system not having net framework like vb6 programs?
No, any program targeting the .Net runtime will require that version of the runtime that it was compiled against. Note that having the 3.0 runtime does not mean an application with the 1.1 runtime will work; it must be the same runtime compiled.
How can you correct syntax logical runtime errors?
How could polymorphism contribute to the evolutionary success of cnidarians in their environment?
Polymorphism occurs when two or more different phenotypes exist in the same population of a species. Polymorphism results from evolutionary processes and contributes to evolutionary success of a species such as cnidarians.
What are the advantages and disadvantages of polymorphism and inheritance?
Polymorphism and inheritance are often treated as being the same thing because you cannot have runtime polymorphism without inheritance. However, you can have inheritance without runtime polymorphism. You can also have compile time polymorphism without inheritance. Inheritance relates to the way in which a new class of object can be derived from an existing class of object. The new class inherits all the public and protected members of the existing class, and thus allows us… Read More